Web Dev Cohort 1.0 is Live!Β π
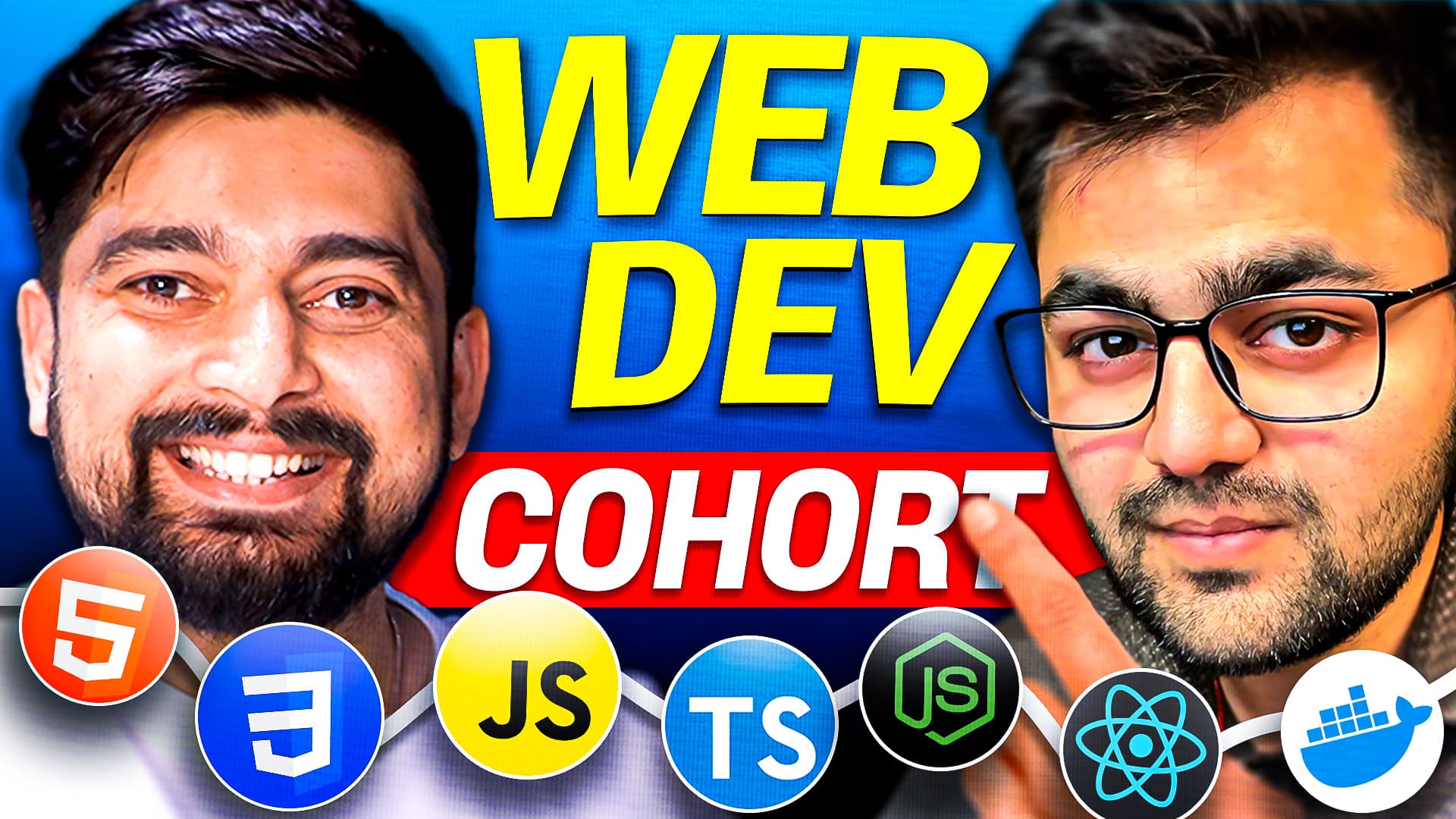
Web Dev Cohort
Master full-stack web development with Web Dev Cohort - Live 1.0.
Learn HTML, CSS, JS, React, Next.js, Node, Docker, databases like MongoDB/PostgreSQL, DevOps with AWS (ECR, EC2, CloudFront),
modern workflows like Turbo Repo, TypeScript, and GitHub CI/CD.
Use coupon code PIYUSH10 for additional 10% OFF π

6 months
Web Warriors βοΈ
Objective: Learn the building blocks of the web, how the internet works, and how the protocols make communication happen.
1. How the Internet Works
- β Introduction to the concept of the Internet
- β Overview of the World Wide Web (WWW) and its connection to the internet
- β How data is transferred across networks
- β Understanding IP addresses, domain names, and routing
- β Key Concepts: Internet Service Providers (ISPs), Routers, DNS
2. DNS Magic and Internals
- β What is DNS (Domain Name System)?
- β How DNS resolves domain names to IP addresses
- β Types of DNS records: A, CNAME, MX, and more
- β DNS Hierarchy: Root DNS servers, TLDs, and authoritative DNS servers
- β How browsers query DNS servers to load websites
- β Key Concepts: Recursive queries, Caching, TTL (Time-to-Live)
3. Server-Client Architecture
- β What is a Client-Server model?
- β Differences between Client and Server in web applications
- β HTTP request-response cycle
- β How browsers act as clients that request resources from web servers
- β Introduction to web servers and web hosting
- β Key Concepts: Web servers (Apache, Nginx), Client-side vs Server-side, Request headers, Response codes
4. Internet Protocols
- β Introduction to network protocols: What are they, and why are they needed?
- β The role of protocols in ensuring data transfer integrity and reliability
- β Overview of key protocols: HTTP, HTTPS, FTP, and SMTP
- β How secure protocols like HTTPS ensure encrypted communication
- β Key Concepts: Protocol stacks, Handshakes, Encryption
5. TCP/IP
- β What is TCP/IP and why it is fundamental for data transmission
- β How TCP ensures reliable data transfer via packet acknowledgment and retransmission
- β The role of IP in addressing and routing data packets across networks
- β Understanding ports and sockets in TCP/IP communication
- β Key Concepts: Three-way handshake, Packet loss, Routing tables
6. UDP (User Datagram Protocol)
- β What is UDP, and how does it differ from TCP?
- β Understanding when and why UDP is used (e.g., in video streaming or gaming)
- β Comparison of TCP and UDP performance (reliability vs speed)
- β Key Concepts: Datagram-based transmission, Low overhead, Connectionless communication
7. TCP Handshakes and 3-Way Handshakes
- β What is a 3-way handshake in TCP?
- β Detailed breakdown of the 3 phases: SYN, SYN-ACK, and ACK
- β The purpose of the handshake: Establishing a reliable connection
- β How data is transmitted after the handshake is complete
- β Key Concepts: Reliable connection establishment, Sequence numbers, Acknowledgments
8. HTTP & HTTPS Protocols
- β Introduction to HTTP (HyperText Transfer Protocol) and HTTPS (Secure version of HTTP)
- β What happens during an HTTP request-response cycle?
- β Understanding status codes: 200 OK, 404 Not Found, 500 Internal Server Error
- β Introduction to SSL/TLS and how it secures data during transmission
- β Key Concepts: Request methods (GET, POST, PUT, DELETE), HTTPS handshake, Certificate Authorities
Spider-Man πΈοΈ
Objective: Learn the foundations of web design by mastering HTML and CSS, creating the structure and styling that powers the web.
1. HTML Basics β The Webβs Skeleton
- β Introduction to HTML (HyperText Markup Language) and its role in web development
- β Understanding HTML tags and elements
- β Building a simple webpage using HTML: html, head, body, header, footer
- β Working with text elements: h1, p, a, ul, ol, li
- β Structuring content with semantic HTML: section, article, nav, main, aside
- β Key Concepts: Elements, Tags, Nesting, Attributes, Semantic HTML
2. HTML Forms and Inputs β User Interaction
- β Creating forms with form, input, textarea, select, button
- β Understanding form submission, GET and POST methods
- β Using input types (text, email, number, password)
- β Validating form data with attributes like required, min, max
- β Key Concepts: Form elements, Input validation, Method types, Accessibility in forms
3. CSS Basics β Styling the Web
- β Introduction to CSS (Cascading Style Sheets) and its purpose
- β Understanding the CSS box model: margin, border, padding, content
- β Styling text, colors, and fonts with color, font-family, font-size, line-height
- β Understanding the concept of specificity and how CSS selectors work
- β Applying styles to HTML elements using selectors, classes, and IDs
- β Key Concepts: Box model, Selectors, Specificity, Inline vs external CSS
4. CSS Layouts β Building Responsive Pages
- β Introduction to layout techniques in CSS: display, position, float, flexbox, grid
- β Building layouts with Flexbox: Aligning items, creating rows and columns
- β Introduction to CSS Grid: Creating complex grid-based layouts
- β Media queries: Making websites responsive to different screen sizes
- β Key Concepts: Flexbox, Grid layout, Responsive design, Mobile-first approach
5. Advanced CSS Styling
- β Using pseudo-classes and pseudo-elements: :hover, :focus, :nth-child
- β Animations and transitions: Making elements move or change on user interaction
- β Styling links, buttons, and forms for better UX
- β Key Concepts: Pseudo-classes, Pseudo-elements, Animations, Transitions
6. CSS Frameworks β Speeding Up Development
- β Introduction to popular CSS frameworks: Bootstrap, TailwindCSS
- β How to use a CSS framework to quickly style pages
- β Customizing and overriding default styles in a framework
- β Key Concepts: Grid systems, Utility-first design, Responsive frameworks
7. HTML5 & CSS3 Features
- β HTML5 semantic elements: header, footer, main, article, section
- β New input types in HTML5: date, email, tel, range, color
- β CSS3 properties: border-radius, box-shadow, gradient, transitions
- β Key Concepts: Modern HTML5 elements, Advanced CSS3 techniques, Cross-browser compatibility
JavaScript Surgeons π§π»ββοΈ
Objective: Master the fundamentals and advanced concepts of JavaScript, as well as the DOM, in scripting dynamic web pages.
1. JavaScript Basics β The Language of the Web
- β Introduction to JavaScript and its role in web development
- β Understanding variables and data types: string, number, boolean, object, array, null, undefined
- β Declaring variables with var, let, const
- β Understanding scope (Global, Local, Block Scope) and hoisting
- β Basic operators: +, -, *, /, %, ==, ===
- β Control flow statements: if, else, else if, switch, ternary operator
- β Key Concepts: Variables, Data Types, Operators, Conditionals
2. JavaScript Functions β Reusable Code
- β What are JavaScript functions? Why are they important?
- β Declaring functions with the function keyword
- β Understanding parameters, arguments, and return values
- β Arrow functions: A modern way to define functions
- β Function scope and closures
- β Key Concepts: Function Declaration, Function Expression, Parameters vs Arguments, Return Value, Scope, Closures
3. JavaScript Loops β Automating Repetitive Tasks
- β Introduction to loops: for, while, and do...while loops
- β Looping through arrays
- β Loop control statements: break and continue
- β Using for...in and for...of loops
- β Key Concepts: Iteration, Loop control, Array iteration
4. JavaScript Arrays β Working with Collections of Data
- β What is an array?
- β Creating arrays, accessing elements by index
- β Array methods: push, pop, shift, unshift, splice, slice, indexOf, etc.
- β Using loops to iterate through arrays
- β Multi-dimensional arrays
- β Key Concepts: Array indices, Array methods, Array iteration
5. JavaScript Objects β Working with Key-Value Pairs
- β What is an object?
- β Creating objects with literal notation and constructor functions
- β Accessing object properties
- β Adding, updating, and deleting object properties
- β Object methods
- β Key Concepts: Object properties, Object methods, Object iteration
6. DOM Manipulation β Interacting with the Webpage
- β Introduction to the DOM (Document Object Model)
- β Selecting elements in the DOM: getElementById, getElementsByClassName, querySelector, querySelectorAll
- β Modifying element content: innerHTML, textContent
- β Modifying element attributes
- β Creating and appending new elements to the DOM
- β Key Concepts: DOM tree, Element selection, Content manipulation, Dynamic HTML
7. JavaScript Events β Responding to User Actions
- β Introduction to events: click, mouseover, keypress, submit, etc.
- β Adding event listeners to elements
- β Event handlers and callback functions
- β Event bubbling and capturing
- β Preventing default event behavior
- β Key Concepts: Event listeners, Event handlers, Event propagation
8. Asynchronous JavaScript β Handling Delays
- β Introduction to asynchronous programming and why it's important
- β Callbacks and callback hell
- β Promises: a better way to handle asynchronous operations
- β Async/Await syntax: A cleaner way to handle promises
- β Making API requests with fetch
- β Key Concepts: Asynchronous operations, Callbacks, Promises, Async/Await
9. ES6+ Features β Modern JavaScript
- β Introduction to ECMAScript 6 (ES6) and beyond
- β Let and const: improved variable declarations
- β Arrow functions: concise function syntax
- β Template literals: string interpolation
- β Destructuring: extracting values from arrays and objects
- β Spread and Rest operators
- β Classes: a more object-oriented approach
- β Modules: organizing code
- β Key Concepts: Modern JS syntax, improved code organization, new language features
10. Working with APIs β Fetching Data from Servers
- β What are APIs?
- β Introduction to RESTful APIs
- β Making API requests with fetch
- β Handling API responses
- β Working with JSON data
- β Key Concepts: API endpoints, HTTP methods, JSON parsing, Asynchronous data fetching
11. Error Handling β Debugging JavaScript
- β What are JavaScript errors?
- β Try...catch statements: handling errors gracefully
- β Throwing custom errors
- β Debugging tools and techniques: console.log, browser debugger
- β Key Concepts: Error types, try...catch, Debugging
12. JavaScript Testing β Ensuring Code Quality
- β Introduction to JavaScript testing
- β Unit testing basics
- β Testing frameworks: Jest, Mocha
- β Writing tests for functions and modules
- β Key Concepts: Unit tests, Test frameworks, Code Quality
13. JavaScript Best Practices and Patterns
- β Code organization and structure
- β Writing clean and maintainable code
- β Design patterns: common solutions to recurring problems
- β Avoiding common mistakes
- β Key Concepts: Code maintainability, Design patterns, Code quality
Node Ninja π₯·
Objective: Master backend development with Node.js, learning how to create powerful server-side applications, handle HTTP requests, and connect with databases.
1. Introduction to Node.js β The Power of JavaScript on the Server
- β What is Node.js and how it differs from traditional server-side languages
- β Understanding the Node.js runtime environment
- β The event-driven, non-blocking I/O model in Node.js
- β Setting up a simple Node.js application
- β Installing and using Node.js with npm (Node Package Manager)
- β Key Concepts: Event-driven architecture, Non-blocking I/O, npm, Modules
2. Understanding the Event Loop β Node.js Architecture
- β What is the event loop and how does Node.js handle concurrency
- β How Node.js uses the event loop to process requests asynchronously
- β Blocking vs Non-blocking code execution
- β The importance of callbacks and promises in managing asynchronous code
- β Key Concepts: Event loop, Asynchronous processing, Callbacks, Promises
3. Creating a Basic HTTP Server
- β How to create a basic HTTP server with Node.js using the `http` module
- β Setting up routes to handle different HTTP requests (GET, POST, PUT, DELETE)
- β Sending and receiving data with the server
- β Working with request and response objects
- β Key Concepts: HTTP server, Request/Response objects, Routing
4. Express.js β Simplifying Backend Development
- β Introduction to Express.js and how it simplifies Node.js backend development
- β Setting up an Express app and defining routes
- β Handling dynamic data with URL parameters and query strings
- β Middleware in Express: What is middleware and how to use it
- β Built-in Express middleware functions (e.g., body-parser, cookie-parser)
- β Key Concepts: Express.js, Routing, Middleware, Request handling
5. RESTful API Design β Building APIs with Express
- β What is a RESTful API and how to structure it
- β Designing endpoints and handling HTTP methods (GET, POST, PUT, DELETE)
- β Using query parameters and request bodies for passing data
- β Returning JSON data and handling status codes in API responses
- β Key Concepts: REST API design, CRUD operations, Status codes, JSON responses
6. Working with Databases β Connecting Node.js to Databases
- β Introduction to databases (SQL vs NoSQL)
- β Using MongoDB with Node.js (Setting up MongoDB, connecting via Mongoose)
- β Working with CRUD operations in MongoDB (Create, Read, Update, Delete)
- β Introduction to SQL databases (using MySQL/PostgreSQL with Node.js)
- β Using ORMs (Object Relational Mappers) like Drizzle, Prisma to interact with SQL databases
- β Key Concepts: MongoDB, SQL, NoSQL, CRUD, Mongoose, Sequelize, ORMs
7. Authentication and Authorization β Securing Your Application
- β Introduction to user authentication and authorization concepts
- β Using JWT (JSON Web Tokens) for stateless authentication
- β Setting up user login and registration endpoints
- β Password hashing with bcrypt.js
- β Role-based access control and securing routes with middleware
- β Key Concepts: Authentication, Authorization, JWT, bcrypt, Role-based access control
8. Working with File Systems β Reading and Writing Files
- β Introduction to the Node.js `fs` module for file system operations
- β Reading files asynchronously and synchronously
- β Writing files to the serverβs file system
- β Handling file uploads (e.g., using `multer` for handling multipart forms)
- β Key Concepts: File system module, File reading/writing, File uploads
9. Building Real-time Applications β WebSockets with Socket.io
- β What are WebSockets and how they enable real-time communication
- β Setting up a WebSocket server using the `ws` module or Socket.io
- β Sending and receiving real-time data between the client and server
- β Use cases for real-time apps (e.g., chat applications, live notifications)
- β Key Concepts: WebSockets, Real-time communication, Socket.io
10. Deploying Your Node.js Application
- β Introduction to deployment options for Node.js applications
- β Deploying Node.js apps on cloud platforms (Heroku, AWS, DigitalOcean, etc.)
- β Setting up environment variables for different environments (production, development)
- β Configuring reverse proxies with Nginx or Apache
- β Key Concepts: Deployment, Cloud services, Reverse proxies, Environment variables
11. API Rate Limiting β Protecting Your Endpoints
- β What is API rate limiting and why it's important
- β Implementing rate limiting to prevent abuse of your APIs (e.g., using `express-rate-limit`)
- β Configuring custom rate limiters for different endpoints
- β Handling rate limit exceeded errors and responses
- β Key Concepts: Rate limiting, Throttling, API protection, express-rate-limit
12. Logging & Monitoring β Tracking Application Health
- β Introduction to logging and monitoring in Node.js applications
- β Using logging libraries like Winston and Morgan for structured logging
- β Setting up logging levels (info, warn, error) and storing logs in files or external services
- β Integrating monitoring tools like PM2 for process management and performance monitoring
- β Key Concepts: Logging, Winston, Morgan, PM2, Monitoring, Application health
13. GraphQL
- β Introduction to GraphQL
- β What is GraphQL and how it differs from REST
- β GraphQL architecture: schema, queries, mutations, and subscriptions
- β Setting up a GraphQL server with Apollo Server
- β Writing simple GraphQL queries and mutations
- β Writing GraphQL Queries, Mutations, and Subscriptions
- β Creating complex queries and mutations
- β Understanding resolvers and schema design
- β Subscriptions for real-time data updates
- β Handling errors in GraphQL
14. Monitoring with PM2
- β Introduction to PM2 for Node.js process management
- β Setting up PM2 for application monitoring
- β Auto-restarting Node.js apps on crashes
- β Monitoring performance with PM2 logs and stats
- β Log rotations
React Alchemist π§π»
Objective: Master the art of building dynamic, interactive, and scalable web applications using React.js, Next.js, Tailwind CSS, ShadCN, and other modern technologies. Learn best practices, performance optimizations, and advanced patterns for building professional-grade React applications.
1. Introduction to React β The Modern JavaScript Library
- β What is React and why itβs the go-to library for building UIs
- β Understanding the virtual DOM and how React improves performance
- β Setting up a React project using `create-react-app` or Vite
- β JSX: A syntax extension for JavaScript that allows writing HTML in JS
- β Rendering elements and basic React components
- β Key Concepts: React, JSX, Virtual DOM, Components
2. Components and Props β The Building Blocks of React
- β Understanding functional and class components
- β Passing data between components using props
- β How to use children and default props
- β Breaking down UI into smaller reusable components
- β Key Concepts: Components, Props, Reusability, State vs Props
3. State Management β React's Core Mechanism
- β Understanding state in React and how it drives component re-renders
- β Managing state within functional components using `useState`
- β Lifting state up to parent components for sharing data
- β Conditional rendering based on component state
- β Key Concepts: State, `useState`, Re-rendering, Lifting state up
4. React Lifecycle Methods β Understanding Component Lifecycles
- β Introduction to component lifecycle in class components
- β Exploring React's lifecycle methods (e.g., `componentDidMount`, `componentWillUnmount`)
- β Using `useEffect` hook for side effects in functional components
- β How Reactβs lifecycle methods help manage data fetching, cleanup, and DOM manipulation
- β Key Concepts: Lifecycle methods, `useEffect`, Mounting, Unmounting, Side effects
5. Event Handling β Interactivity in React
- β Handling events like clicks, form submissions, and user input
- β Binding event handlers in React components
- β Using `event.preventDefault()` and `event.stopPropagation()` for event flow control
- β Creating controlled and uncontrolled form components
- β Key Concepts: Event handling, Forms, `event.preventDefault()`, `event.stopPropagation()`
6. React Hooks β Bringing Functionality to Components
- β Introduction to React Hooks and their importance in functional components
- β Using `useState` for state management and `useEffect` for side effects
- β Exploring other hooks: `useContext`, `useReducer`, `useCallback`, `useMemo`
- β Best practices for working with hooks
- β Key Concepts: Hooks, `useState`, `useEffect`, `useContext`, `useReducer`, `useCallback`
7. React Router β Navigating Between Pages
- β Introduction to React Router for client-side routing
- β Setting up React Router for multiple views (pages) in a single-page application (SPA)
- β Using `Link` and `Route` to navigate between components
- β Dynamic routing with URL parameters and query strings
- β Key Concepts: React Router, `Link`, `Route`, Dynamic routing, SPA
8. State Management with Context API β Global State for Your App
- β What is the Context API and when to use it for global state management
- β Creating a context, providing it, and consuming it in components
- β Using `useContext` to access and update global state
- β Avoiding prop drilling with the Context API
- β Key Concepts: Context API, Global state, `useContext`, Prop drilling
9. Forms in React β Building Dynamic Forms
- β Controlled vs uncontrolled forms in React
- β Handling form submissions and form validation
- β Building complex forms with multiple input fields
- β Using third-party libraries like Formik or React Hook Form for easier form management
- β Key Concepts: Forms, Controlled inputs, Validation, Formik, React Hook Form
10. Styling in React β From CSS to Styled Components
- β Styling React components using traditional CSS, CSS Modules, and styled-components
- β Introduction to CSS-in-JS libraries like Emotion and styled-components
- β Managing responsive designs in React apps
- β Best practices for CSS architecture in React (BEM, CSS Modules)
- β Key Concepts: CSS, CSS-in-JS, styled-components, Responsive design
11. Performance Optimization β Making Your App Fast
- β Understanding Reactβs rendering behavior and performance bottlenecks
- β Techniques to optimize performance in React apps (e.g., memoization, lazy loading)
- β Using React's `React.memo`, `useMemo`, and `useCallback` hooks
- β Code splitting and lazy loading with React Suspense
- β Key Concepts: Performance, Memoization, `React.memo`, `useMemo`, `useCallback`, Code splitting
12. Deploying Your React Application β Going Live
- β Deployment options for React apps: Netlify, Vercel, Heroku, AWS, etc.
- β Configuring environment variables for different deployment environments
- β Building the React app for production using `npm run build`
- β Setting up continuous deployment for automatic updates
- β Key Concepts: Deployment, Continuous integration, Production build, Environment variables
13. React Advanced Patterns β Enhancing Your Skills
- β Introduction to higher-order components (HOCs) and render props
- β Understanding compound components for reusable logic
- β Custom hooks: Creating your own hooks for code reuse
- β Using context providers and consumers for state management
- β Key Concepts: Higher-order components, Render props, Custom hooks, Compound components
14. Building Scalable React Applications β Architecture and Design
- β Structuring large-scale React applications using component-based design
- β Organizing components, hooks, and utilities for maintainability
- β Breaking the application into features for better scalability
- β Using state management tools like Redux or Zustand for advanced state management
- β Key Concepts: Scalability, Component-based design, Architecture, Redux, Zustand
15. NextJS - The React Framework for Full-Stack Applications
- β Introduction to Next.js and why itβs an essential tool for React developers
- β Setting up a Next.js project and understanding its file-based routing
- β Static Site Generation (SSG) and Server-Side Rendering (SSR) in Next.js
- β API Routes in Next.js for backend functionality within a React app
- β Dynamic routing and how Next.js handles URL parameters
- β Key Concepts: Next.js, File-based Routing, SSR, SSG, API Routes
Deploy and AI Squad π
Objective: Master the deployment of web applications to the cloud, ensuring scalability, security, and high availability. Learn about cloud infrastructure using AWS services like EC2, ECS, CloudFront, Load Balancers, Docker, and more to bring applications from development to production. After that we will move towards AI. What is AI, AI powered applications, Vector Databases (Pinecone etc), RAG based systems, Image background removal, image generation, text summarization and creative ideas with AI
1. Introduction to Cloud Deployment β The Basics of Scaling Apps
- β What is cloud deployment and why itβs essential for modern applications
- β Overview of different cloud providers: AWS, Azure, Google Cloud
- β Understanding high availability, scalability, and fault tolerance in the cloud
- β Benefits of cloud computing in terms of flexibility, cost, and performance
- β Key Concepts: Cloud deployment, Scalability, High availability, Fault tolerance
2. AWS EC2 β Virtual Servers in the Cloud
- β What is Amazon EC2 (Elastic Compute Cloud)?
- β Setting up an EC2 instance to host your application
- β Understanding EC2 instance types, regions, and availability zones
- β Connecting to EC2 instances using SSH and setting up security credentials
- β Key Concepts: EC2 Instances, Regions, Availability Zones, SSH
3. Configuring EC2 Security Groups β Controlling Access to Your Instance
- β What are security groups and why they are critical for security?
- β Setting inbound and outbound rules for EC2 instances
- β Restricting access to your EC2 instance using security group configurations
- β Best practices for EC2 security group management
- β Key Concepts: Security groups, Inbound and outbound rules, Port management
4. Load Balancers β Ensuring High Availability and Reliability
- β What is a Load Balancer and why itβs important for scalability and availability?
- β Introduction to Elastic Load Balancing (ELB) in AWS
- β Configuring an Application Load Balancer (ALB) for HTTP/HTTPS traffic
- β Setting up health checks to monitor instance health
- β Key Concepts: Load Balancing, Application Load Balancer (ALB), Health checks
5. AWS CloudFront β Content Delivery Network for Faster Load Times
- β Introduction to AWS CloudFront and why itβs crucial for performance optimization
- β Configuring CloudFront distributions to serve static assets (images, scripts, stylesheets)
- β Understanding caching, edge locations, and how CloudFront speeds up content delivery
- β Integrating CloudFront with your S3 bucket for static website hosting
- β Key Concepts: CloudFront, Caching, Edge locations, Content delivery
6. Docker β Containerization for Consistency and Portability
- β Introduction to Docker and its benefits for application deployment
- β Creating Docker images and running containers locally
- β Understanding Dockerfiles and how to write them for your application
- β Setting up multi-container applications using Docker Compose
- β Key Concepts: Docker, Containers, Dockerfiles, Docker Compose
7. AWS ECS β Elastic Container Service for Running Docker Containers
- β What is AWS ECS and why itβs used for deploying Docker containers?
- β Setting up an ECS cluster to run Docker containers on EC2 instances
- β Creating ECS tasks and services to manage containerized applications
- β Configuring ECS with Application Load Balancer (ALB) for traffic distribution
- β Key Concepts: ECS, Containers, ECS Tasks, ECS Services, ALB
8. AWS ECR β Elastic Container Registry for Storing Docker Images
- β What is Amazon ECR and how it works with ECS and Docker?
- β Pushing and pulling Docker images to/from Amazon ECR
- β Securing your ECR repository with IAM permissions and access control
- β Best practices for managing container images in ECR
- β Key Concepts: ECR, Container registry, IAM, Pushing and pulling Docker images
9. Target Groups β Directing Traffic to the Right Containers
- β What are Target Groups and how do they work with Load Balancers?
- β Configuring Target Groups in AWS to route traffic to ECS services
- β Setting up health checks for Target Groups to ensure only healthy containers receive traffic
- β Understanding the concept of weighted routing and path-based routing in Target Groups
- β Key Concepts: Target Groups, Routing, Health checks, Weighted routing
10. Security Rules & IAM Roles β Managing Permissions for Security
- β Introduction to AWS Identity and Access Management (IAM) for managing permissions
- β Setting up IAM roles and policies to grant permissions to EC2, ECS, and other services
- β Best practices for securing your AWS infrastructure using IAM
- β Configuring VPC security groups and network ACLs to restrict access
- β Key Concepts: IAM, Roles, Policies, Permissions, VPC Security Groups, Network ACLs
11. Scaling and Auto Scaling β Adjusting Resources Based on Demand
- β Introduction to Auto Scaling and how it helps scale EC2 instances based on demand
- β Setting up Auto Scaling groups with EC2 instances and configuring scaling policies
- β Using ECS Auto Scaling to scale Docker containers in response to traffic spikes
- β Key Concepts: Auto Scaling, Scaling policies, Load balancing, Scaling EC2 and ECS
12. Continuous Deployment (CI/CD) β Automating the Deployment Pipeline
- β Introduction to CI/CD pipelines and why theyβre essential for modern web apps
- β Setting up CI/CD with GitHub Actions
- β Automating Docker container builds and deployment to ECS/ECR
- β Rolling updates and blue/green deployments with ECS for zero downtime
- β Key Concepts: CI/CD, Code Pipeline, Code Build, GitHub Actions, Docker deployment, Blue/Green deployments
13. Monitoring and Logging β Keeping Your Application Healthy
- β Introduction to AWS CloudWatch for monitoring EC2, ECS, and other resources
- β Setting up CloudWatch alarms for resource utilization and application health
- β Using AWS Cloud Trail for logging and auditing API calls in your AWS account
- β Integrating logging libraries (e.g., Winston, Morgan) into your Docker containers
- β Key Concepts: CloudWatch, Monitoring, Cloud Trail, Logging, Winston, Morgan
14. Cost Optimization β Managing Your AWS Resources Efficiently
- β Best practices for managing AWS costs and avoiding unnecessary expenses
- β Using AWS Trusted Advisor and Cost Explorer to monitor and optimize costs
- β Setting up AWS Budgets and alerts to track spending
- β Key Concepts: Cost optimization, AWS Budgets, Trusted Advisor, Cost Explorer
15. Security Best Practices β Keeping Your Application Secure in the Cloud
- β Setting up Web Application Firewalls (WAF) to protect against common attacks
- β Using SSL/TLS certificates for secure communication in your application
- β Regularly auditing IAM roles and security policies to minimize the risk of unauthorized access
- β Securing Docker containers and images with scanning tools and security patches
- β Key Concepts: Security, WAF, SSL/TLS, Docker security, IAM auditing
Frequently asked questions
We are here to help you with any questions you may have. If you don't find what you need, please contact us at piyushgarg.dev@gmail.com